The blog post outlines how I set up my environment to run C code in VSCode.
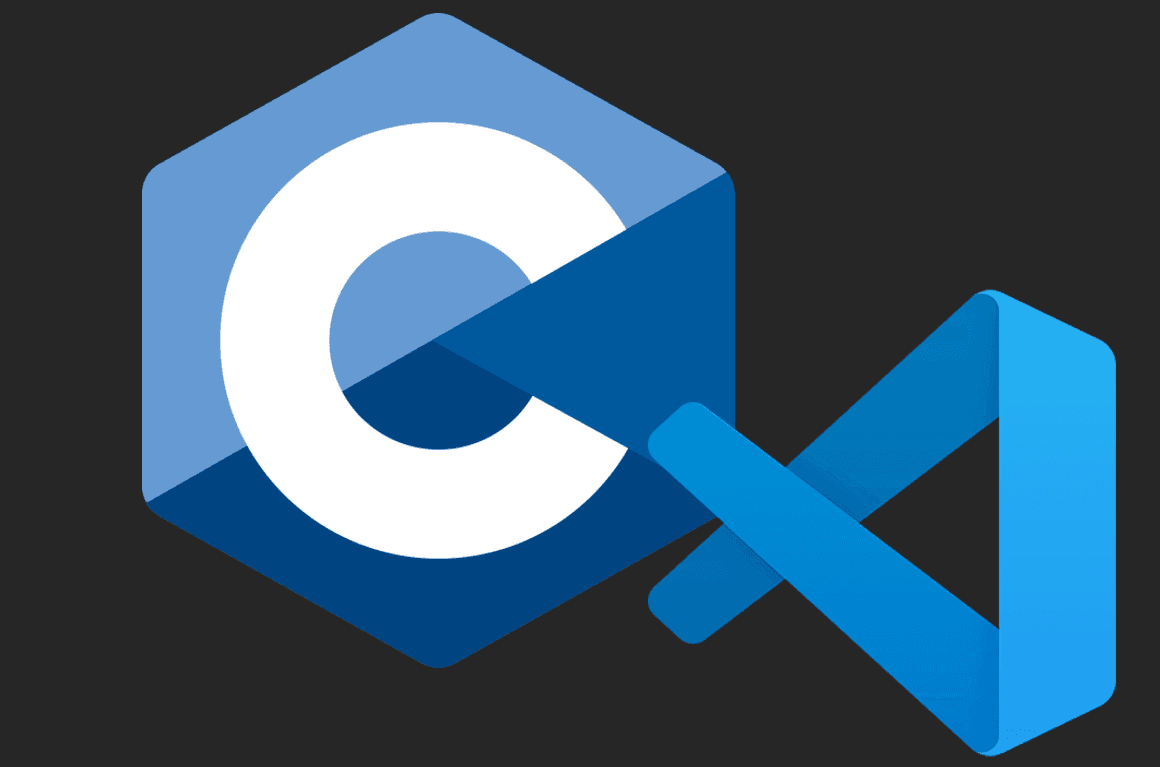
Before diving into the code, you will need to set up your environment first. There are multiple ways to set it up, but I would like to highlight how I did it on my device (M2 MacBook).
Install Clang
To run a C program, you will need a corresponding compiler. There are multiple compilers like Clang and GCC, but I would recommend Mac users to use Clang.
clang --version
You can put the above script in your terminal to check if you have your compiler installed already. If there is a error saying things like clang doesn't exist, then you will have to run the following in your terminal.
xcode-select --install
After running the above command, make sure you have it installed by running clang --version
again.
Set Up VSCode
If you don't have VSCode installed, go to this link and set it up. This is many developers' favorite IDE, with countless useful features and extensions. (Use Dark Mode for your eyes.) In VSCode, open the Extensions page and type "C" in the search box. At the top, there is an official extension from Microsoft named "C/C++" so install and enable that extension. Upon installing, they might ask you to set up your C++ environment. Choose clang compiler and you will be good to go.
Also, open the Extensions page again and type "code runner" in the search box. You will find a extension "Code Runner" by Jun Han, so make sure you install and enable that extension as well. This will come in handy for executing and debugging your code. Once you installed Code Runner, I would highly recommend going to settings of Code Runner extension and turn on "Run on Terminal".
Write Your First C Code
To test if you have configured the environment correctly, let's write a simple code and run it. Create a file called hello.c in VSCode, and type the following:
#include <stdio.h>
int main() {
printf("Hello, World!");
return 0;
}
The above code simply prints "Hello, World!" in your terminal. (Don't worry about the syntax and details for now.) Now, save the code by pressing "Ctrl+S" and press the play button at the top right corner. (This might ask you to select between clang and gcc. Select clang and continue.)
After the build succeeds, you should see "Hello, World!" in your Terminal. Congratulations! You have successfully set up your environment and run your first C program!
Making Changes
Now that you've set things up correctly, making changes to your code and seeing it reflect on your output is straight forward. let's say to add your name instead of "World," first rewrite it in hello.c:
#include <stdio.h>
int main() {
printf("Hello, TK!"); // Changed from "World" to "TK"
return 0;
}
After saving the change with "Ctrl+S", click the toggle button next to the play button, and select "Run Code". You should see the output on your Terminal. Congratulations! You are all set to embark on the journey to becoming a C programmer!