The blog post introduces the very basics of C.
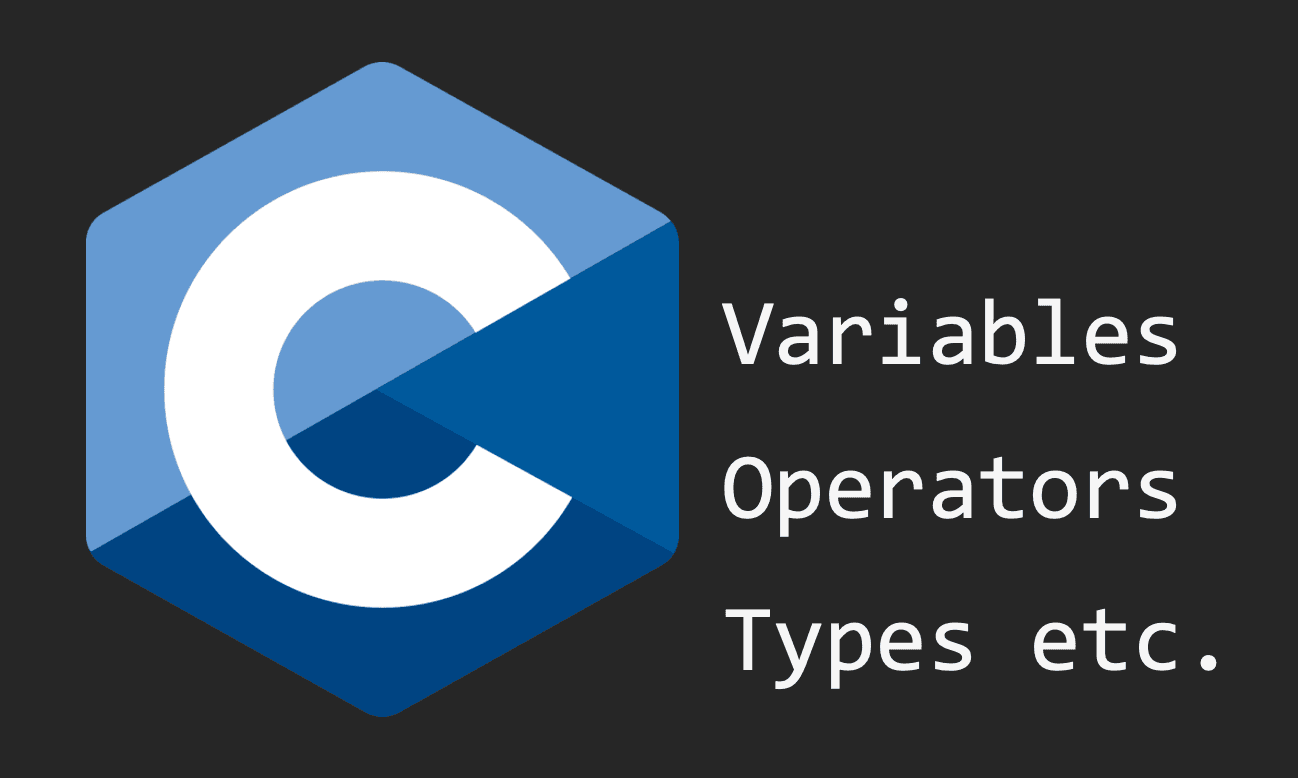
Variables
Let's get right into the coding. When you want to assign a value to a variable in C, you can do so as follows:
type variableName; // Declaration
variableName = value // Initialization
// Examples
int a;
a = 123;
char b;
b = 'b';
In C, you need to declare the type you want to use for that variable to decide how much memory should be allocated to it. You do not have to write the declaration and initialization separately.
int c = 1;
Make sure that variable names are as descriptive as possible. When we want to display the value stored
in a variable with printf
, we use format specifiers corresponding to that data type.
// For int
printf("C: %d", c);
The %
sign is a placeholder, and d
(decimal) is a format specifier for int
.
Data Types
Here, let's see what kind of data types exist in C.
Type | Usage | Format Specifier | Side Note |
---|---|---|---|
Single Character | char a = 'A'; or signed char a = 1; | %c or %d | It can store integer of 1 byte (-128 to 127). If %c is used for integer stored in char, corresponding character from ASCII table will get displayed. |
Array of Characters | char a[] = "Hey"; | %s | You can specify the size of array by putting number in []. (char a[10] = "Hey";) |
Short Integer | short int a = 1; or short a = 1; | %d | It uses 2 bytes of memory (-32,768 to 32,767). |
Integer | int a = 1; or long int a = 1; | %d | It uses 4 bytes of memory (-2,147,483,648 to 2,147,483,647). If unsigned , the format specifer becomes %u . |
Long Integer | long long int a = 1; | %lld | It uses 8 bytes of memory (-9 quintillion to 9 quintillion). If unsigned , the format specifer becomes %llu . |
Floating Point | float a = 3.14; | %f | It uses 4 bytes of memory (32 bits of precision or 6-7 digits). |
Double | double a = 3.1415926535; | %lf | It uses 8 bytes of memory (64 bits of precision or 15-16 digits), double the memory of float . |
Boolean | bool a = true; | %d | You will need to use include <stdbool.h>; to display a boolean. false corresponds to 0 while true corresponds to 1. |
In addition to the above, we have the unsigned
syntax, which only stores positive numbers.
For example, unsigned char
stores values from 0 to 255 instead of -128 to 127. You can put unsigned
in front of short
, int
, long
, and long long int
, each of which has its corresponding format specifiers.
When you store a number larger than it can handle, it overflows and goes back to the smallest number
it can store. Therefore, you need to be aware of how much memory and range of values each data type can store.
Format Specifier
The format specifier can specify many things about how the numbers get displayed.
double pi = 3.1415926535;
// Specifying decimal point %.[decimal point]
printf("Pi: %.2lf\n", pi); // => Pi: 3.14
// Specifying field width %[field width]
printf("Pi: %8.2lf\n", pi); // => Pi: 3.14
printf("Pi: %8.3lf\n", pi); // => Pi: 3.141
printf("Pi: %8.4lf\n", pi); // => Pi: 3.1415
// Left align %-
printf("Pi: %-8.2lf\n", pi); // => Pi: 3.14
printf("Pi: %-8.3lf\n", pi); // => Pi: 3.141
printf("Pi: %-8.4lf\n", pi); // => Pi: 3.1415
As you can see from the above examples, you can specify decimal point to display, field width, and alignment.
Constants (const
vs #define
)
Sometimes, you want to have a value that cannot be modified during execution, like . When we want to define constant in C, we can do the following:
const float PI = 3.14;
If we try changing PI
after initializing with const
, we will get an error. The naming convension is
to use capital letters for the constants. There is actually an alternative for achieving a similar thing,
which is #define
.
#define PI 3.14
The difference is that #define
replaces every PI
with 3.14
in the code before compilation, while const
is
treated just like a variable during compilation. Because of this, #define
is not type-safe and might lead
to unexpected behavior when you have PI
in places you did not intend to replace with 3.14
. Hence, in most
cases, it is preferred to use const
.
Arithmetic Operators
In C, these are the arithmetic operators available.
// + Addition
// - Substitution
// * Multiplication
// / Dibision
// % Modulus
// ++ Increment
// -- Decrement
int x = 5;
int y = 2;
int z = x + y; // z=7
int z = x - y; // z=3
int z = x * y; // z=10
int z = x / y; // z=2
x++; // x=6
y--; // y=1
One odd thing we notice here is that we get z=2 for int z = x / y;
.
Let's change the type of z
to float so that we can see more decimal points.
float z = x / y // z=2.000000
Why is it still 2? It is because integer division truncates decimals. We need
to use convert either of x
or y
to float
.
float z = x / (float) y // z=2.500000
There are some cases when you want to perform calculation and update the value of variable.
Instead of doing x = x * 2
or x = x - 1
, you can do the following.
int x = 5;
x += 2; // x=7
x -= 2; // x=5
x *= 2; // x=10
x /= 2; // x=5
x %= 3; // x=2
Math Functions
Here, I will introduce some of the useful predefined math functions you can use in C.
#include <math.h>
// Square root
double a = sqrt(9); // a=3.0000
// Exponent
double b = pow(2, 4); // b=2^4=16
// Rounding
int c = round(3.14); // c=3
// Ceil
int d = ceil(3.14); // d=4
// Floor
int e = floor(3.99); // e=3
// Absolute Value
double f = fabs(-10); // f=10
// Logarithm
double g = log(3); // g=1.1
// Trigonometry (in radians)
double h = sin(1); // h=0.84
double i = cos(1); // i=0.54
double j = tan(1); // j=1.56
User Input
Finally, let's talk about how we can take user input in C. You can do the following to retrieve user input.
// Declare variable to store user input to
char name[25];
printf("Your Name: ");
scanf("%25s", name); // User Input
printf("Hello, %s!", name);
int age;
printf("Your Age: ");
scanf("%d", &age); // User Input
printf("You are %d years old!", age);
If you run this, you should be able to provide user input to the code.
However, you might also realize that if you put space in your name like
"Micheal Jordan", It only prints "Hello, Micheal!". It is because
scanf
only reads up to a whitespace. To have a whitespace in your
user input, we can use fgets
instead.
#include <string.h> // You need to import string.h
char name[25];
printf("Your Name: ");
fgets(name, 25, stdin); // User Input with Whitespace
name[strlen(name)-1] = '\0';
printf("Hello, %s!", name);
The above will print "Hello, Micheal Jordan!" if "Micheal Jordan" is provided. (Don't worry about the details for now.)
Exercises
From this article, there will be an exercise section where you can test your understanding of the material introduced in the article. I highly recommend solving these questions by yourself after reading the main part of the article. You can click on each question to see its answer.
Resources
- Bro Code. 2022. C variables 💰. YouTube.
- Bro Code. 2022. C data types 📊. YouTube.
- Bro Code. 2022. C format specifiers 🔧. YouTube.
- Bro Code. 2022. C constants 🚫. YouTube.
- Bro Code. 2022. C arithmetic operators ➗. YouTube.
- Bro Code. 2022. C augmented assignment operators 🧮. YouTube.
- Bro Code. 2022. C user input ⌨️. YouTube.
- Bro Code. 2022. C math functions 📚. YouTube.