The blog post is about strings in C.
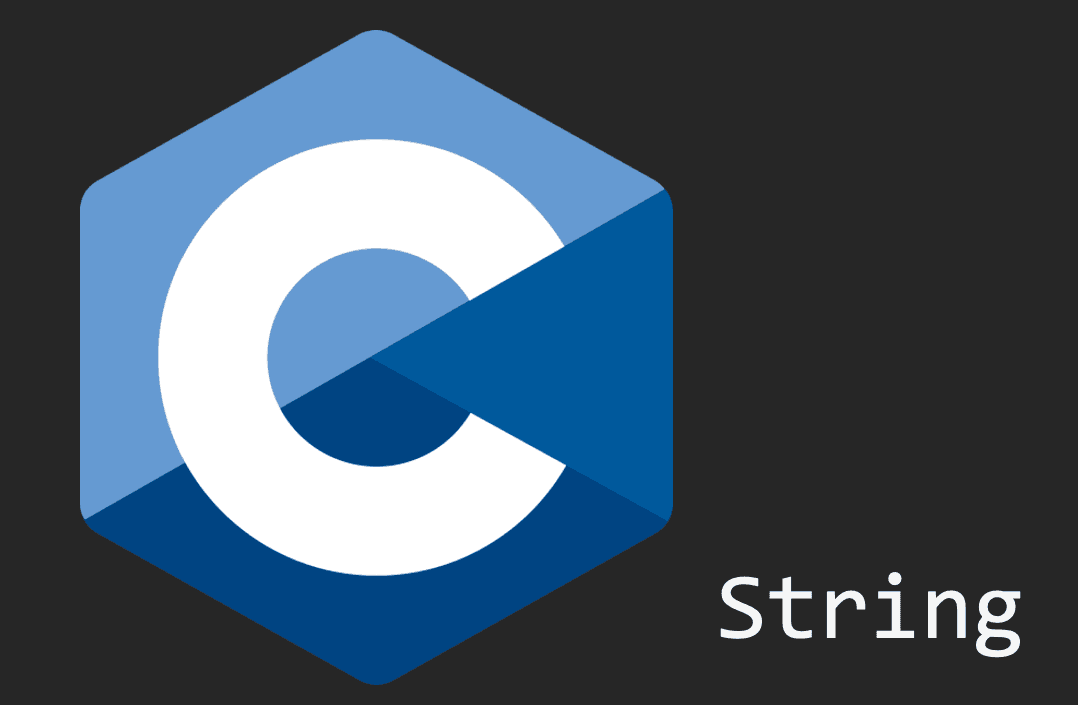
In C, a string is handled a little bit differently than in other higher-level languages. Hence, this article focuses on understanding how strings work in C.
Functions for Strings
Since a string is just an array of chars in C but is still used extensively, there are various predefined functions for dealing with strings. The following shows some examples of useful predefined functions.
// Package with predefined functions for strings
#include <string.h>
char firstName[] = "TK";
char lastName[] = "Blog";
// Convert a string to lower or upper case
strlwr(lastName); // converts to lowercase (blog)
strupr(lastName); // converts to uppercase (BLOG)
// Concatenate two strings
strcat(firstName, lastName); // concatenate lastName to the end of firstName (TKBlog)
strncat(firstName, lastName, 1); // concatenate n characters of lastName to the end of firstName (TKB)
// Copy a string to another
strcopy(firstName, lastName); // copy lastName to firstName (firstName = Blog)
strncopy(firstName, lastName, 2); // copy n characters of lastName to firstName (firstName = Bl)
// Set the content of a string
strset(firstName, '?'); // set all characters of firstName to ? (firstName = ??)
strnset(firstName, '?', 1); // set first n characters of firstName to ? (firstName = ?K)
// Reverse a string
strrev(lastName); // reverse lastName (lastName = "gloB")
// Get the length of a string
int l = strlen(lastName); // returns string's length as int (l = 4)
// String Comparisions
int res = strcmp(firstName, lastName); // compare all characters of strings (0 if same, other numbers if not)
int res = strncmp(firstName, lastName, 2); // compare n characters of strings (0 if same, other numbers if not)
int res = strcmpi(firstName, lastName); // compare all characters of strings ignoring case (0 if same, other numbers if not)
int res = strnicmp(firstName, lastName, 2); // compare n characters of strings ignoring case (0 if same, other numbers if not)
There are many other predefined functions, but we will need to learn the concept of pointers first to fully understand them, so we will skip them for now.
WARNING: If you have the same set up as mine, there are many functions like strlwr
, strupr
, strcmpi
, etc. that
you cannot use because they are not part of the standard C library string.h
.
2D Arrays
When we define an array of arrays, or a 2D array, we can do so like below.
int matrix[2][3] = {{1,2,3},{4,5,6}};
You can change the number of the array and display as follows.
matrix[0][1] = 7; // set the value of 0th row, 1th column to 7
// Calculate the number of rows and columns with sizeof
int rows = sizeof(matrix) / sizeof(matrix[0]);
int cols = sizeof(matrix[0]) / sizeof(matrix[0][0]);
// Display matrix with nested loop
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
printf("%d ", matrix[i][j]);
}
printf("\n");
}
// => 1 7 3
// 4 5 6
Array of Strings
Why did I even talk about 2D arrays when the article is on strings? It is because an array of strings is 2D array of chars.
char names[][10] = {"Drake", "Kendrick", "J Cole"};
Just like a normal 2D array, you can change a character of the strings like below.
names[0][1] = 'a'; // => set 1th character of 0th string to a (Drake -> Daake)
However, you cannot change an entire string in an array with =
. You need to use strcopy
function.
Also, you do not need nested for loops for displaying an array of strings.
strcpy(names[0], "Adonis"); // set 9th string to "Adonis" (Drake -> Adonis)
for (int i = 0; i < sizeof(names)/sizeof(names[0]); i++) {
printf("%s\n", names[i]);
}
// => Adonis
// Kendrick
// J Cole
Swapping
When you want to swap values of two variables, you have to set up new variable temp
for temporarily storing
the value of the variable that gets assigned a value of another variable first.
int x = 5;
int y = 4;
int temp;
temp = x; // x=5, y=4, temp=5
x = y; // x=4, y=4, temp=5
y = temp; // x=4, y=5, temp=5
However, as we have seen from the example of array of strings, we cannot use =
for assinging new string. You
need to use strcpy
function when swapping strings.
char x[] = "TK";
char y[] = "Blog";
char temp[15];
strcpy(temp, x); // x=TK, y=Blog, temp=TK
strcpy(x, y); // x=Blog, y=Blog, temp=TK
strcpy(y, temp); // x=Blog, y=TK, temp=TK
However, you should be careful when using strcpy
function because having shorter string for the second argument
can lead to unexpected behavior. You can prevent this by using the same size for both x
and y
by defining them as
char x[15] = "TK"; char y[15] = "Blog"
.
Exercises
From this article, there will be an exercise section where you can test your understanding of the material introduced in the article. I highly recommend solving these questions by yourself after reading the main part of the article. You can click on each question to see its answer.
Resources
- Bro Code. 2022. C string functions 🔠. YouTube.
- Bro Code. 2022. C 2D arrays ⬜. YouTube.
- Bro Code. 2022. C array of strings🧵. YouTube.
- Bro Code. 2022. C swap values of two variables 🥤. YouTube.