The blog post introduces the concept of namespaces in C++.
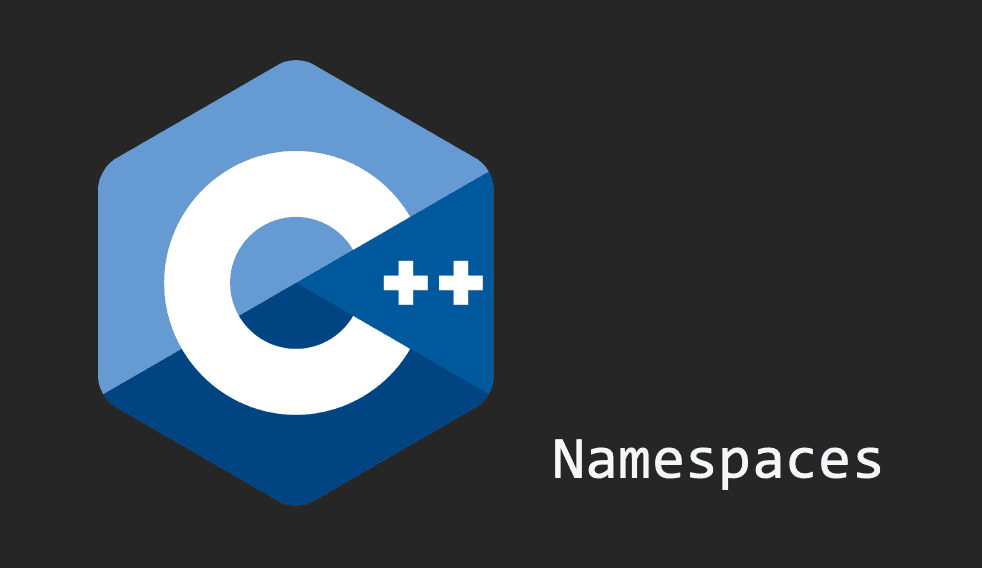
So far, we have been using using namespace std
in every C++ code without
explaining what it means. In this article, we will finally discuss namespaces
and their purpose.
Namespaces
In C++, you cannot use the same name for functions with the same input and output types,
as this creates ambiguity. For example, the code below results in ambiguity regarding the
definition of the print
function.
#include <iostream>
using namespace std;
void print() {
cout << "Print1" << endl;
};
void print() {
cout << "Print2" << endl;
};
This issue might seem easy to spot, but it can occur frequently as you use more modules and libraries. Hence, the best practice in C is to use the module name as a prefix for a function to minimize naming conflicts. In C++, the concept of namespaces allows us to manage names, as shown below.
using namespace std;
namespace print1 {
void print() {
cout << "Print1" << endl;
};
};
namespace print2 {
void print() {
cout << "Print2" << endl;
};
};
int main () {
print1::print(); // => Print1
print2::print(); // => Print2
return 0;
};
You can use the ::
operator, also known as the scope resolution operator, to specify
the namespace and the function. This helps avoid naming conflicts. Alternatively, you can
use using namespace
to specify which namespace to use by default within a given scope.
using namespace print1;
int main () {
print(); // => Print1
print2::print(); // => Print2
return 0;
};
This is why using namespace std
sets the namespace of the standard library, allowing you
to avoid using the scope resolution operator for every cout
and endl
call. Additionally,
you can define a namespace within another namespace and access the inner namespace with
using namespace outer::inner
.
Why using namespace std
Is a Bad Practice
While we have been using using namespace std
, it is generally considered bad practice.
This is because the std
namespace contains numerous function names, which can restrict
our ability to choose names freely. For instance, the standard library's algorithm
header
contains a min
function, which might conflict with a custom min
function that we define.
#include <iostream>
#include <algorithm>
using namespace std;
template <class T>
const T& min(const T& a, const T& b) {
return a;
}
int main() {
cout << "Min: " << min(4, 5) << endl; // Err!
return 0;
}
Although such conflicts are easy to spot in smaller examples, it becomes exponentially
harder to avoid these ambiguities as the codebase grows. The problem is compounded when
a header file uses using namespace std
, as the content of the header file is copied
into the source code via the #include
macro, potentially polluting the namespace.
#include <iostream>
#include <algorithm>
#include "library.h" // which uses `using namespace std;`
template <class T>
const T& min(const T& a, const T& b) {
return a;
}
int main() {
cout << "Min: " << min(4, 5) << endl; // Err!
return 0;
}
In the example above, while the code does not directly use using namespace std
,
including a header file like #include "library.h"
introduces ambiguity. The issue becomes
hard to detect, especially in large projects where others might be working on library.h
.
To address this, the best practice is to limit using namespace std
to a local scope or
to specify individual functions within the namespace, such as using std::cout
.
Conclusion
In this article, we discussed the concept of namespaces and the potential problems
associated with using namespace std
. Whether in C or C++, it is important to follow
best practices when designing modules and libraries to avoid naming conflicts.
In C, this involves using prefixes, while in C++, it involves careful use of namespaces.
Resources
- Portfolio Courses. 2023. Namespaces | C++ Tutorial. YouTube.
- Portfolio Courses. 2023. Why 'using namespace std' Is A Bad Practice | C++ Tutorial. YouTube.