The blog post introduces the very basics of C++.
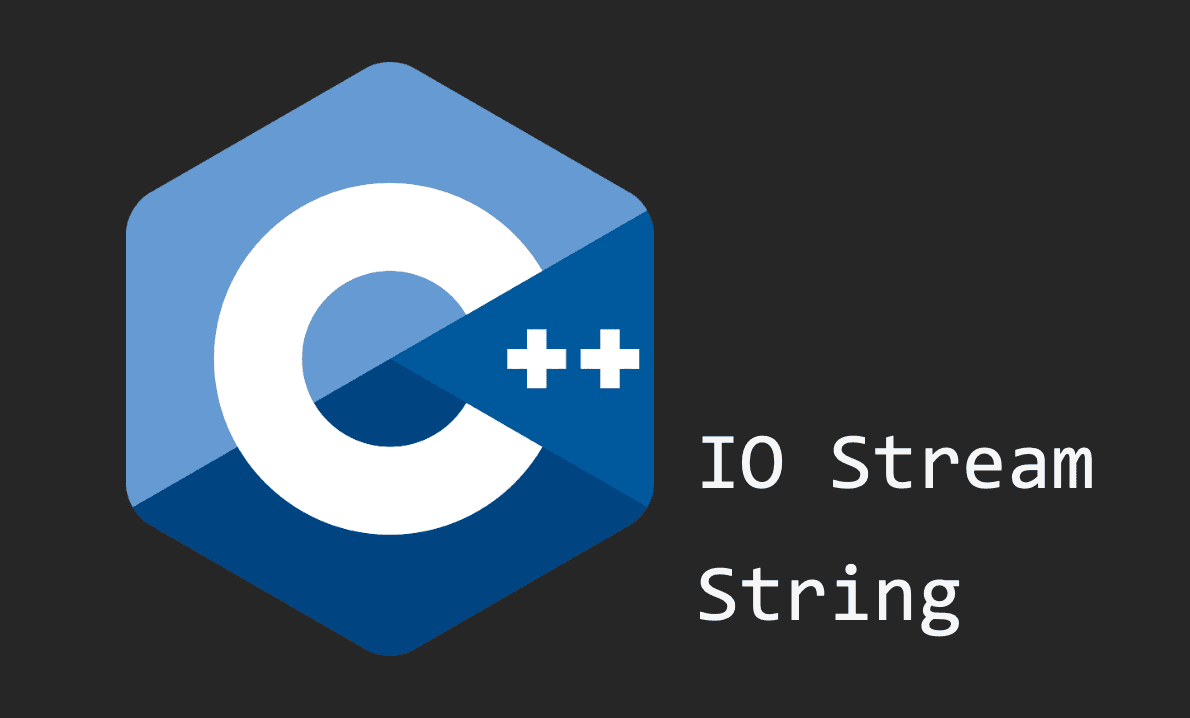
Before We Start
As I mentioned in the previous article, C++ is an extension of C, and thus many of the syntax and functionalities of C are compatible with C++. In this article on the basics of C++, I will focus on some basic functionalities that were not available in C. If you haven't already, I recommend checking out the C series. The environment setup for C++ is the same as for C, so if you haven't set up your environment yet, refer to the article Road to C Programmer #0 - Setting Up Environment. For C++, we use the file name extension ".cpp" and compile the code with the "g++" command instead of "gcc."
IO Stream
Standard streams are preconnected communication channels for input and output (IO) between a
program and its environment. There are three IO connections: standard input (stdin), standard
output (stdout), and standard error (stderr). The printf
function in C is just a wrapper
function that writes formatted strings to the stdout stream. (This explains what the IO monad
is for in Haskell.) In C++, we can still use the printf
function, but we can also use the following:
#include <iostream>
using namespace std;
int main () {
cout << "Hello World" << endl;
return 0;
}
using namespace std
allows us to access features related to standard streams in the iostream
library. (I will cover namespaces in the future.) cout
corresponds to console output, which
can output values of any type to the console. <<
is a text insertion operator that can be
used to chain operations. endl
indicates the end of the sentence, equivalent to \n
.
You can accept user input as shown below:
#include <iostream>
using namespace std;
int main () {
float x;
cout << "Enter a float: ";
cin >> x;
cout << "You entered " << x << endl;
return 0;
}
cin
(console input) takes input from the console, ignoring preceding whitespaces, and >>
is the
stream extraction operator. The trailing whitespaces and text after them are disregarded. If we
fail to cast the input to the declared type of the variable, cin
outputs 1. Hence, we can guard
against the failure like this:
#include <iostream>
using namespace std;
int main () {
float x;
cout << "Enter a float: ";
if (cin >> x) {
cout << "You entered " << x << endl;
}
else {
cin.clear();
cin.ignore(numeric_limits<streamsize>::max(), '\n');
cout << "Invalid Input" << endl;
}
return 0;
}
cin.clear()
is used to clear the error state of the input buffer.
cin.ignore()
ignores the maximum stream size
until it encounters \n
, so the subsequent cin
won't extract invalid input.
There are many other functions in the iostream
library, but the above are the
very basics you must know.
String
In C, a string is just a character array, and we have learned how dangerous strings
can be in terms of memory safety. To make the developer experience a little better,
C++ has a string
library that exposes a string
object.
#include <iostream>
#include <string>
using namespace std;
int main () {
string x = "abcd";
cout << "x: " << x << endl;
cout << "size: " << x.size() << endl; // size of text = 4
cout << "length: " << x.length() << endl; // length of text = 4
cout << "x at 3rd index: " << x[3] << endl;
cout << "x at 3rd index: " << x.at(3) << endl;
cout << "x 3 characters from 1st index: " << x.substr(1, 3) << endl;
x += "efgh";
cout << "x: " << x << endl;
x.append("ijkl");
cout << "x: " << x << endl;
if (x.empty()) {
cout << "x is empty." << endl;
} else {
cout << "x is not empty." << endl;
x.clear();
cout << "x is now empty." << endl;
}
return 0;
}
The above shows some methods available for the string
object. You can obtain the length
of
the string using size
or length
(no more sizeof
!), access a character or substring using at
, []
, or substr
,
and append a string with the +
operator or append
. You can also check if a string is empty
with empty
and clear the string using clear
. It utilizes OOP to make these helpful functions
available in C++. The library also has the following functions to convert types to and from strings:
#include <iostream>
#include <string>
using namespace std;
int main () {
string numberStr = to_string(3.14);
double number = stod(numberStr);
return 0;
}
The string
library offers a function helpful when taking user input with whitespace in between, getline
.
#include <iostream>
#include <string>
using namespace std;
int main () {
string fullname;
cout << "Enter your full name: " << endl;
getline(cin, fullname);
cout << "Hello, " << fullname << endl;
return 0;
}
If you use cin >> fullname
and type in a full name with whitespace, like "Shohei Ohtani," it will
ignore "Ohtani" after the whitespace. getline
allows the text after the whitespace to be captured.
Exercises
From this article, there will be an exercise section where you can test your understanding of the material introduced in the article. I highly recommend solving these questions by yourself after reading the main part of the article. You can click on each question to see its answer.
Resources
- Portfolio Courses. 2022. Console Output With cout Basics | C++ Tutorial. YouTube.
- Portfolio Courses. 2022. User Input With cin Basics | C++ Tutorial. YouTube.
- Portfolio Courses. 2022. Basics Of Using The string Type | C++ Tutorial. YouTube.